Added custom highlight function for nhl-235
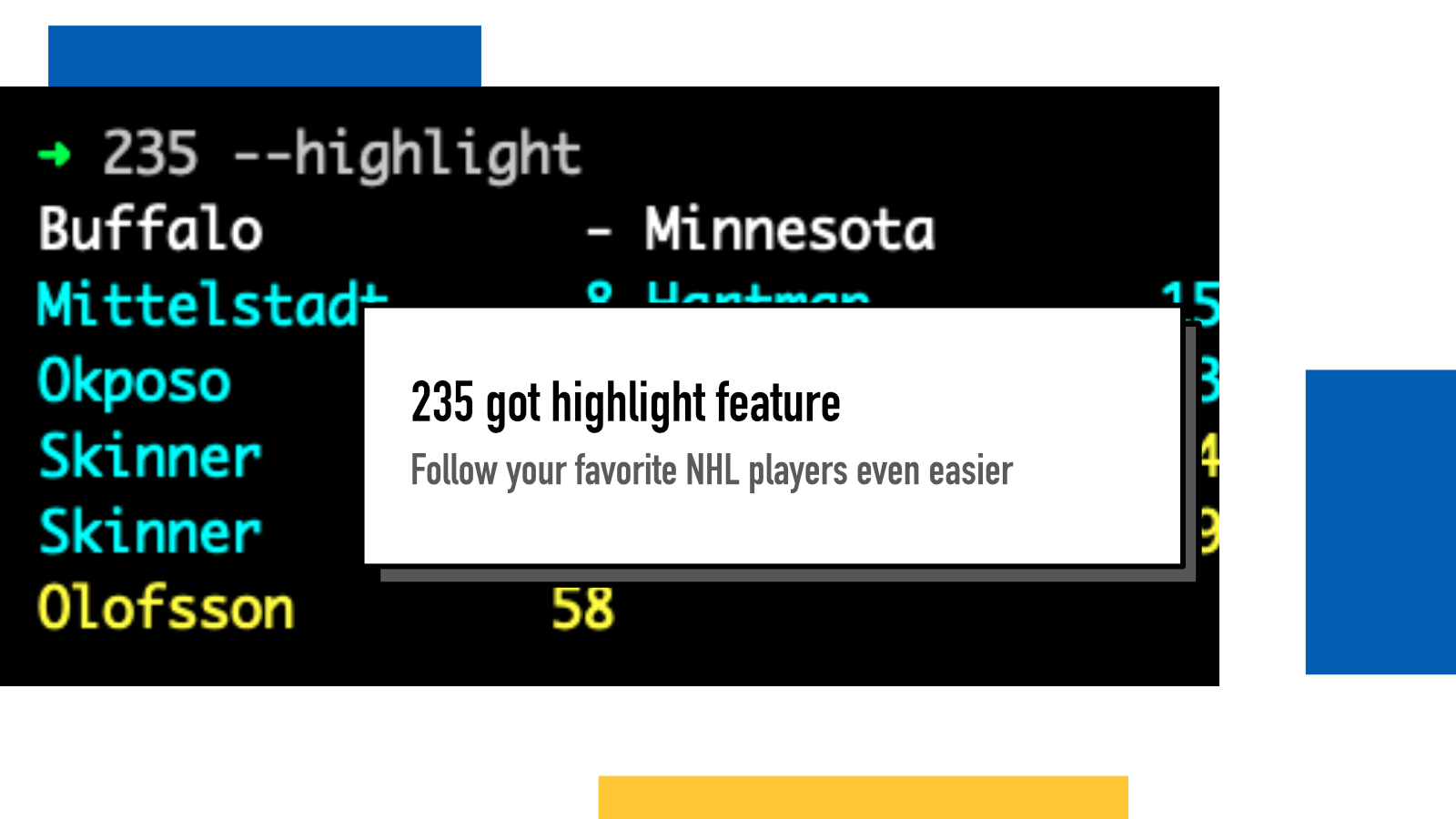
When I originally started developing my nhl-235 project in the beginning of 2021, it was heavily inspired by the teletext service Finnish Broadcasting Company YLE had been running for decades – and promptly named after it as well.
One key feature that I didn't implement (even though I wanted to), was to mimic the way the original highlights Finnish players' points so it's always easy to see on a glance how my favorite players are doing. The main reason for not being able to implement it, was that there was no nationality information in the scores data the API provided and I didn't want to manually maintain a list of players that would always be out of date as players come and go.
Finally last week I got an idea that in retrospective seems so obvious I can't understand why it never occurred to me before, during this past 1+ years that I've developed and used the application. So last weekend I released version 1.2.0 that allows the user to create a custom list of players who they want to highlight and by using a --highlight
flag with the tool, to enable this feature.
Custom highlights
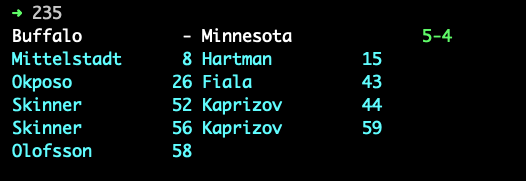
235
without the highlight optionWhen you run 235
without any options, the default colors are cyan for regular goals and magenta for shootout winner.
To enable the highlight, first you need to create a configuration file into your home folder called .235.config
and add there one player (last name only) per line the players you want to highlight, as shown below.
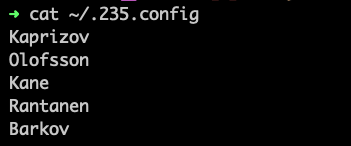
It's a very basic version currently as it only works on the basis of last names and for some players who share their last name with others, you'll get false positives. For me, this was a trade-off I was willing to have since I still know where my favorite players play so it doesn't really bother me if I'll see some extras highlighted.
To highlight the results, you need to run 235 --highlight
and you'll get your favorite scorers highlighted in yellow:
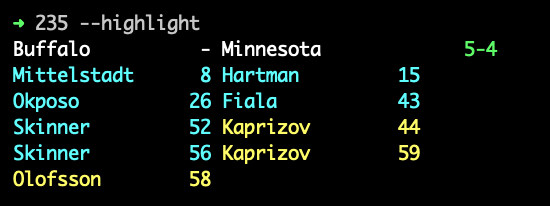
Looking under the hood
Since this project is my learning project in coding Rust, I want to share a few thoughts about developing this feature as well.
First of all, my last release before this was in November so it's been over 4 months since I've written any Rust code so I felt really rusty (no pun intended) to get started again and it felt like everything was so difficult to remember how to do.
I decided to use dirs
crate to make my home folder pathing OS agnostic.
// StdError here is std::io::Error
fn read_highlight_config() -> Result<Vec<String>, StdError> {
// home_dir() is dirs::home_dir
let mut config_file = home_dir().unwrap();
config_file.push(".235.config");
let mut file = File::open(config_file.as_path())?;
let mut contents = String::new();
file.read_to_string(&mut contents)?;
let highlights: Vec<String> = contents
.split('\n')
.map(str::to_string)
.filter(|s| s != "")
.collect();
Ok(highlights)
}
As I've written before, handling things in Option
s and Result
s has been a bit of a challenge and especially now after months of not writing Rust and dirs::home_dir()
returning Option<PathBuf>
, I had more problems with figuring out when to unwrap and so on than I care to count.
Finally I was able to create the function above that reads the config file and returns a vector of the names. I then pass that vector from my main function down to the printing functions and added a new if/else branch to see if a scorer was in the highlights vector and then print it in yellow if it was:
if home.special {
magenta_ln!("{}", message);
} else if highlights.contains(&home.scorer) {
yellow_ln!("{}", message);
} else {
cyan_ln!("{}", message);
}
Future considerations
There are a couple of things I might look into in future:
- Using full names of players to narrow down false positives
- Making the config file more config-y and allow configuring things like setting default flags for highlights and future features so users don't have to always use a flag or set a custom alias (which is my current workaround)
- Using a config file management library like confy if I end up implementing #2. For now though, reading a list of names manually is good enough.
Install the newest version of 235 now
You can install the newest version of 235 either from Rust's crates system with cargo install nhl-235
or by downloading binaries directly from project's GitHub page.
If you're using 235, I'd love to know so don't hesitate to either tweet at me or email me at juhamattisantala at gmail dot com.
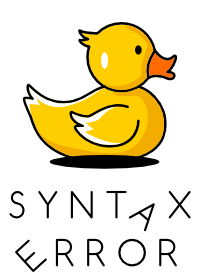
Sign up for Syntax Error, a monthly newsletter that helps developers turn a stressful debugging situation into a joyful exploration.