How to sort a Pokemon deck list?
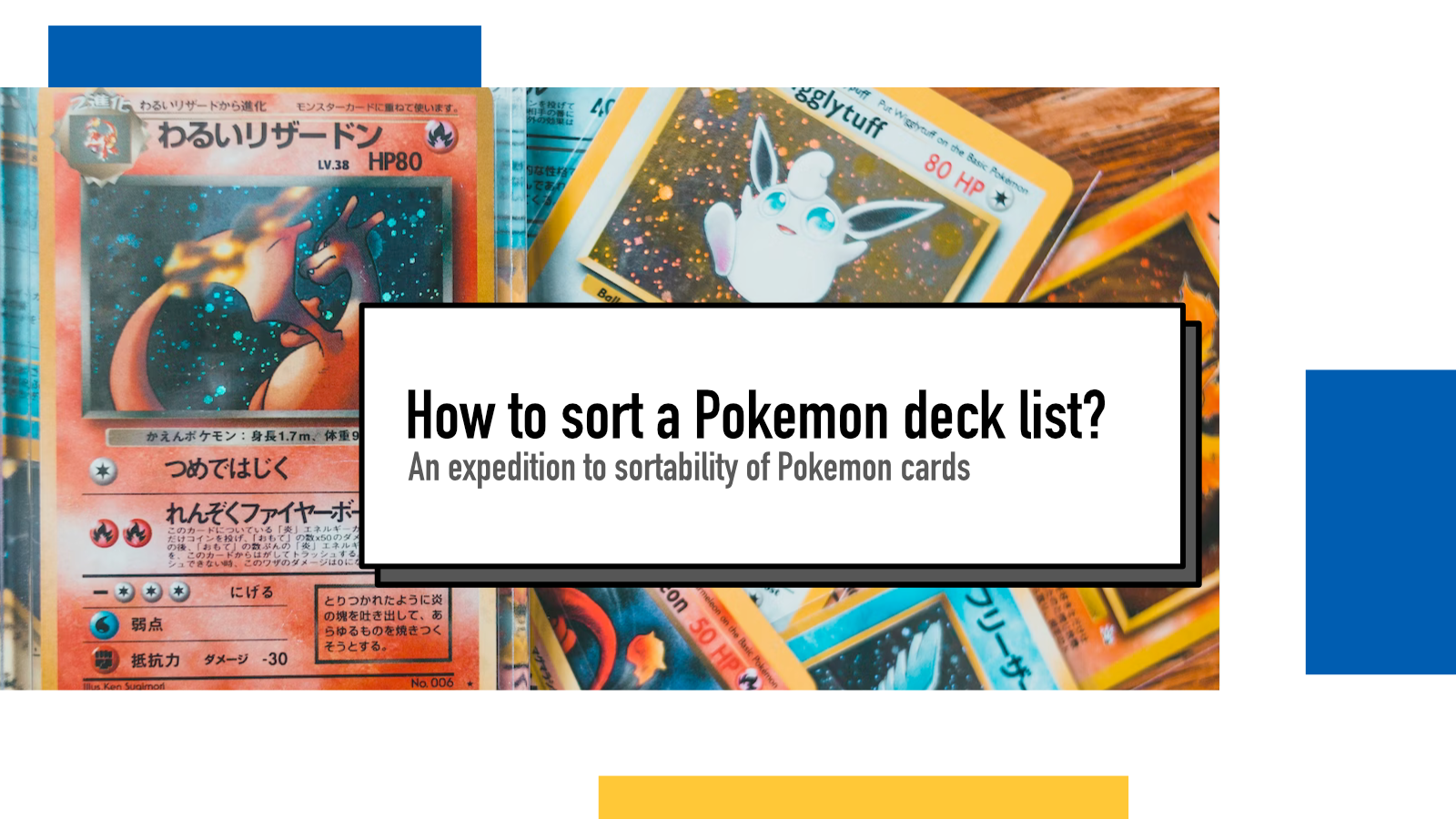
As a developer, sorting an array or list boils down to two things: a sorting algorithm and a sorting criteria. For day-to-day sorting, you don't usually need to worry about the algorithm part – you just use the built-in tools that come with the language unless you specifically need something different.
Over the Easter holiday (a luxurious 6-day holiday for me thanks to the 4-day work week) I started writing code for a new project of mine that I've been designing and experimenting ideas with for a while now. I'm building an online deck builder for Pokemon TCG community. I want to build a world-class experience of building decks for everyone: digital players who don't want to struggle with existing digital tools, content creators who want to showcase their decks in their videos and streams, competitive players who want to quickly iterate their decks and print proxies for quick play testing. More of that project later though, it's only bare bones so far.
The elements of a Pokemon deck
A Pokemon deck is (usually) 60 cards consisting of different types of cards used to play the game. You have your Pokemon cards that are the main stars, your Trainer cards that help you navigate the game and fulfill your strategy and Energy cards that power up your Pokemon to do their fighting.
These get further subdivided: Energies come in two flavors, Basic and Special. Trainers have even more subcategories: Items, Supporters, Tools, Stadiums, Trainers, Goldenrod Game Corner Cards and so on. Pokemon come in different stages and variants: Baby, Basic, Stage 1, Stage 2, BREAK, ex, EX, GX, V, VMAX, VStar and the list goes on.
In addition to these categories, there are other properties that could be used for sorting: cards come in sets, each card (excluding basic energies) have a number within the set, there's also Pokedex numbering that isn't really a TCG property but ties together the Pokemon in-universe in a way that the other properties might not always do.
Pokemon cards also evolve from each other. A Charmander becomes a Charmeleon that becomes a Charizard – and this happens across the sets so that relation transcendes all the other properties.
There's (at least) one more aspect but let's start with these and I'll reveal it in at the end.
What are players expecting?
I'm in a good position because I don't have to invent something novel out of the blue. People have been building decks and sharing decks for decades so there's a lot of precedence.
Supertypes (Pokemon/Trainer/Energy)
One thing that I've found almost universally common is that the main order is: Pokemon, then Trainers, then Energies. The only places where I've seen this order changed is when people lay out their decks in real life for a picture to share. And even then, it's often Pokemon, then Trainers, and finally Energies go wherever there's space on the mat. Which I'll consider to be the same as "last". I don't think a deck has ever been truly identified by its energy.
The official deck list form also uses this order which is probably one reason it's so prevalent.
Whew, we got one criteria down. I'll assign sorting weights to these supertypes: const supertypeSort = { Pokemon: 1, Trainer: 2, Energy: 3 }
and compare those in my custom sort function. Smaller the weight, the earlier it comes in the list.
Subtypes of Energies
When dividing the cards within their supertype, I consider energies to be the easiest: there's usually only a handful of energy types in a given deck and there doesn't seem to be any consistency between different mediums on how to sort them so I'm sorting them basics first, special then. const energySort = { Basic: 1, Special: 2 }
. After that, we can sort the specials and basics alphabetically by their name within their respective groups.
I'm making an assumption here that the order of energies doesn't matter much to the players. Luckily, it's an assumption that can easily be reverted by changing the numbers around if feedback shows that players wish to have their special energies first.
Subtypes of Trainers
Next up, we can look at Trainers as the next easiest one. Let's see how different tools do it currently.
Pokemon has two official digital clients. Pokemon TCG Online – or PTCGO amongst friends – that is being sunset as we speak and Pokemon TCG Live – or Live amongst friends – that is taking the reign as the main platform to play Pokemon digitally.
Pokemon TCG Online
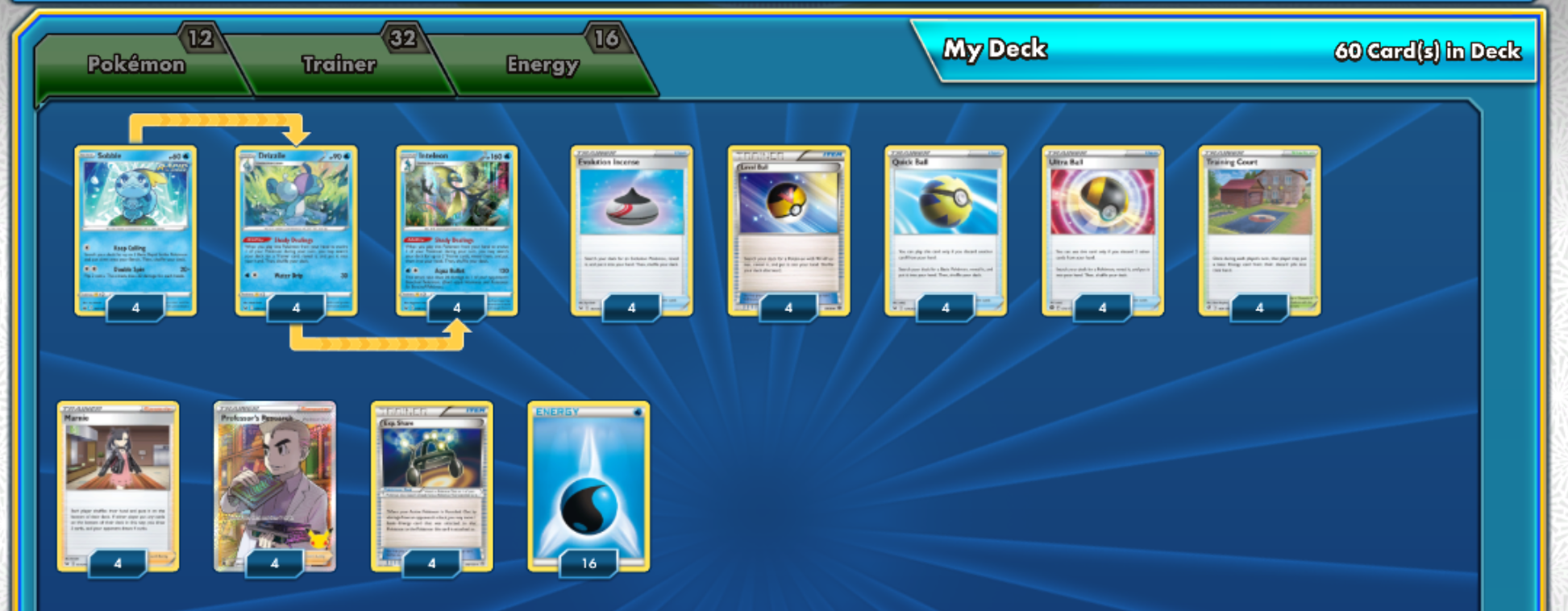
Online starts with Items, follows it up with Stadiums, then Supporters and finally Tools.
Pokemon TCG Live
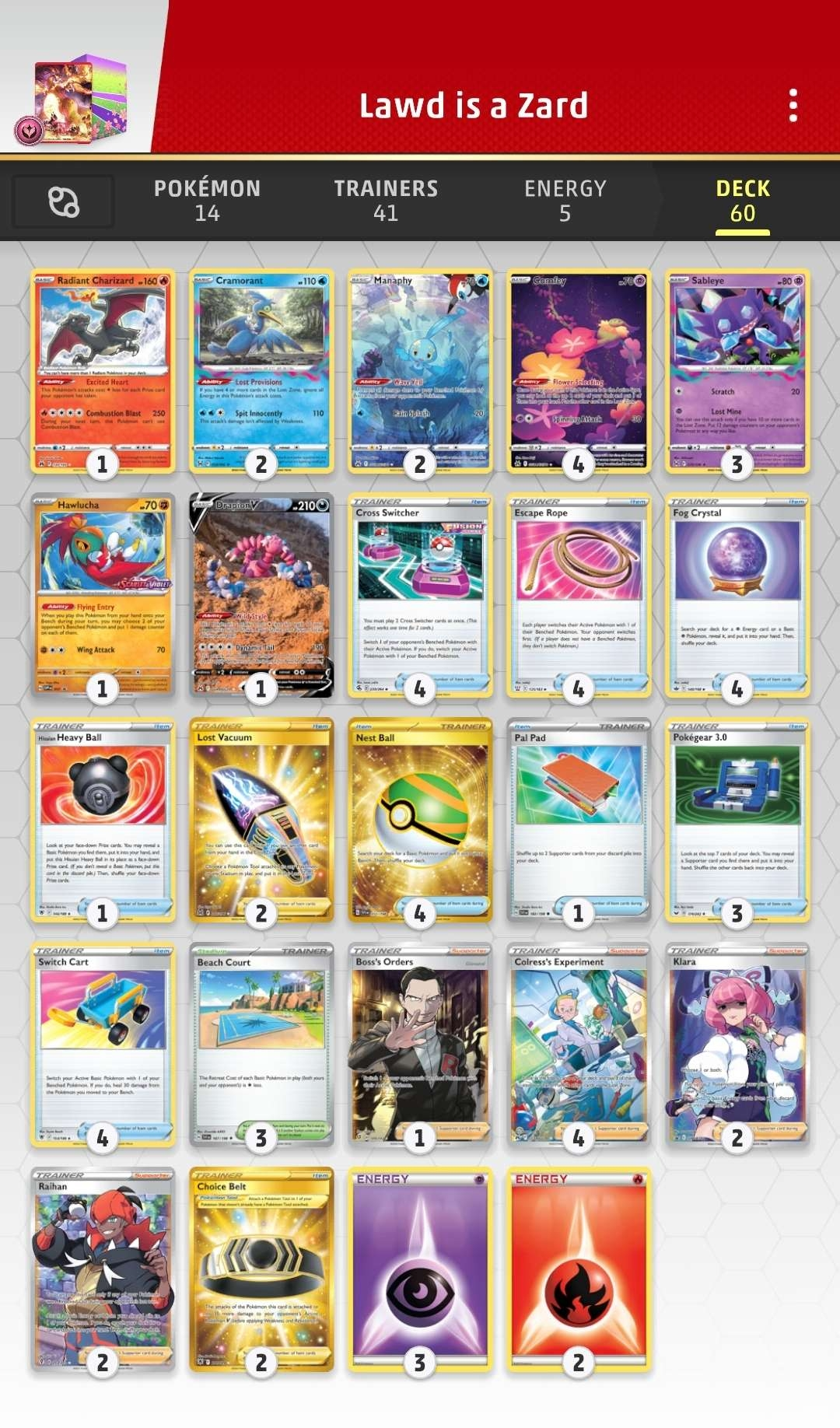
Live sorts Trainers as follows: Items, Stadiums, Supporters, Tools, being the same order as with Online which is not a big surprise given both of these are Pokemon's own tools.
LimitlessTCG.com
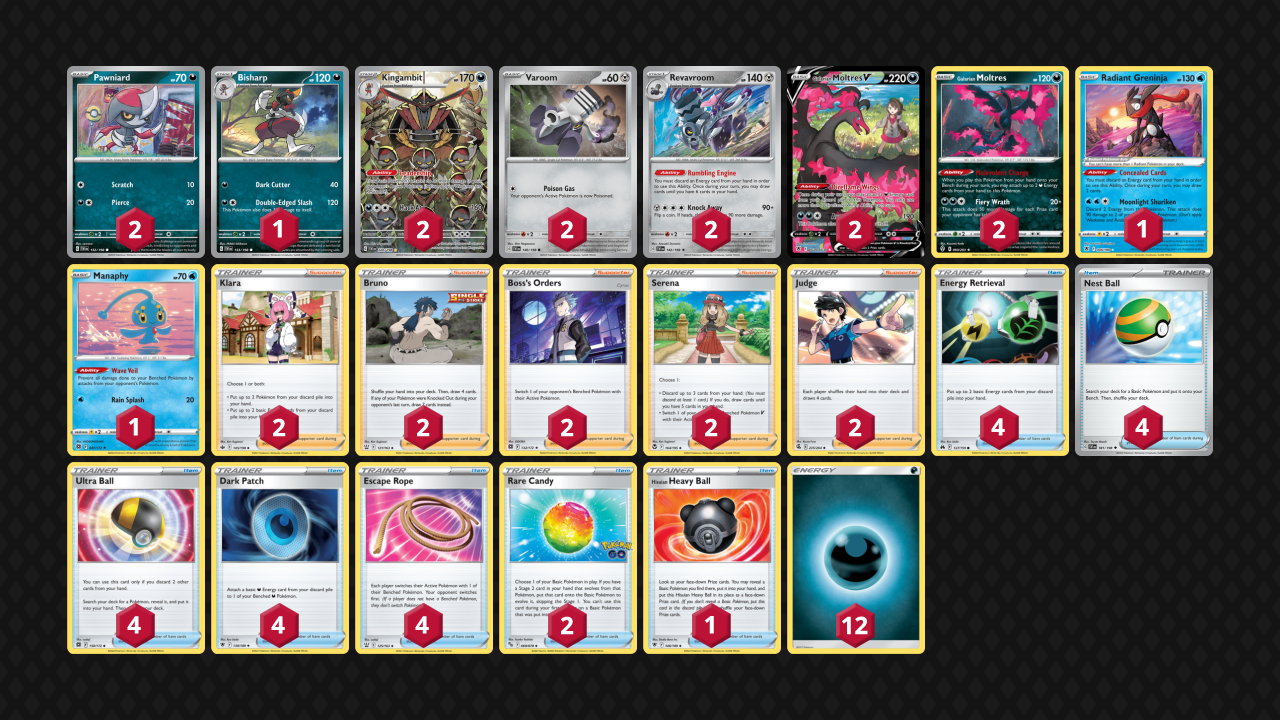
A popular Pokemon TCG resource site LimitlessTCG.com has an image generator tool that takes in a decklist and creates a shareable PNG image from it. It sorts Supporters first, then Items, Tools and finally Stadiums. That is very different from what Pokemon's tools have decided to do.
Physical deck images from the community
As I looked through pictures of decklists shared by the community in different Pokemon TCG Discord servers and social media, I noticed that there's no strong "one order fits all" unwritten rule here. Some people like to start with their supporters, others leave those to the end.
For now, I'm gonna go with the Items (and Item-like cards that follow similar rules of "use as many times as you want"), Supporters, Tools and Stadiums for now.
const trainerSort = {
Item: 1,
Supporter: 2,
"Pokemon Tool": 3,
Stadium: 4
}
The hardest one: Pokemon
We still have one main category left: how do we sort Pokemon. The thing with trainers and energies is that they are pretty much on the same level with each other. A Professor's Research might not be much more important to a deck than a Boss's Orders for example.
That's not the case with Pokemon. There are a few really interesting aspects of the Pokemon cards that make this sorting a bit more challenging.
Evolution lines
Firstly, you want to keep evolution lines together. If you play Charmander, Charmeleon and Charizard, it's quite crucial to have those next to each other to improve the readability of the deck. But you can't look at two cards in isolation and say whether one of them is part of an evolution line in the deck or not. (You can even play evolution cards even without all of their pre-evolutions in the deck.)
A card knows whether it evolves from/to and which card it evolves from/to so the knowledge is there. I just basically need to figure out a way to get that "outside" information into the sorting. One way to do this is to group the cards into subcategories based on their evolution lines. At that point, we wouldn't be sorting individual cards but evolution lines. Instead of deciding if Charmeleon should go before Noivern, we consider if the Charmeleon line (including potentially Charmander and Charizard) should go before Noivern line (including potentially Noibat).
Mains & supports
Second, there's a mental categorization of Pokemon cards into "main attackers" and "support" Pokemon that is deck specific and cannot be automatically deduced from the cards. One deck's supporter Pokemon may be another deck's main card.
This is something that I belive cannot be determined purely based on the data of the cards.
I haven't yet implemented this into the application but my plan is to allow the player to reorder the Pokemon in a way that stays with the deck through automatic sorting. That would allow the player to easily keep an eye on their important main lines when building a deck but also communicate their deck to people when sharing the deck to their community.
Other than that, the sorting pretty much goes the Pokemon types (Grass, Water, Fire, etc) in alphabetical order and then in alphabetical order based on the Pokemon's name within their type.
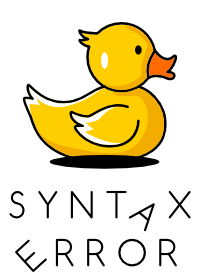
Sign up for Syntax Error, a monthly newsletter that helps developers turn a stressful debugging situation into a joyful exploration.