What's with all these sorts, PHP?
A few days back I was ranting to my friends about PHP arrays. I’m not as much of a PHP hater as many but there’s still some things that really bug me. Everything to do with arrays is one of them. Let’s have a look. I use the phrase normal array to mean a non-associative array (like Python’s list or Java’s array).
Associative ordered arrays
As the documentation states, “An array in PHP is actually an ordered map. A map is a type that associates values to keys. This type is optimized for several different uses; it can be treated as an array, list (vector), hash table (an implementation of a map), dictionary, collection, stack, queue, and probably more.”
I think it’s doing way too much and is way too confusing. You can kinda use it as dozen of different things but not really. If you just define $arr = array(1,2,3)
, it acts like a normal array (what you would call as a list in python). But only as long as you don’t delete anything. unset($arr[1])
removes the second value (2 in this case) and it stops working as a normal array.
$arr = array(1,2,3);
var_dump($arr, json_encode($arr));
unset($arr[1]);
var_dump($arr, json_encode($arr));
The first one encodes into json array [1,2,3]
and the latter one into JSON object {"0": 1, "2": 3}
. So instead of numeral, continuous indices, it converts into associative-only array with two string keys. At least for me it has caused so much confusion when dynamically manipulating these arrays.
But what about sorting?
The PHP standard library is well known for having inconsistent naming and parameter order for functions that do same kind of things. For sorting, there are dozen functions, named consistently but really bad. There’s of course sort
. Instead of giving a flag or parameter to sort to reverse the order, you have rsort
. Then if you want to keep the key=>value pairs intact, there’s asort
(which has caused me most confusions ever since I accidentally used that one instead of sort) and arsort
. Then you can sort with keys ksort
and reverse krsort
. For natural sorting there’s natsort
and case insensitive natcasesort
. And when all this is not enough, you can use custom defined comparing function with usort
, uasort
and uksort
. And the inconsistently named array_multisort
for multidimensional arrays.
Some of the effects you can achieve by using flags with different sorts and for some things, you must use flags since there are no aliases for everything.
I have to admit, I’ve burnt myself too many times with sorting issues on PHP by using the wrong function. Especially when I was just beginning PHP years ago, I just googled and stackoverflowed for answers and would end up with different answers. So I used asort
in one place and usort
elsewhere and didn’t really know the difference.
Too many sorts
I just think there are too many ways to do one thing. It’s confusing for beginners, it is redundant for skilled developers and the naming scheme is – even if consistent – pretty horrible. I am the kind of developer who values ease of writing code over everything else. I don’t really care about efficiency (until it’s absolutely necessary) nor even the benefits of strong static typing. I just want to write some code and enjoy the process.
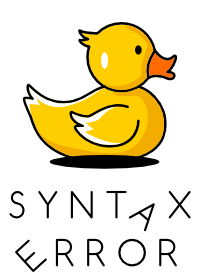
Sign up for Syntax Error, a monthly newsletter that helps developers turn a stressful debugging situation into a joyful exploration.