Document outdated components
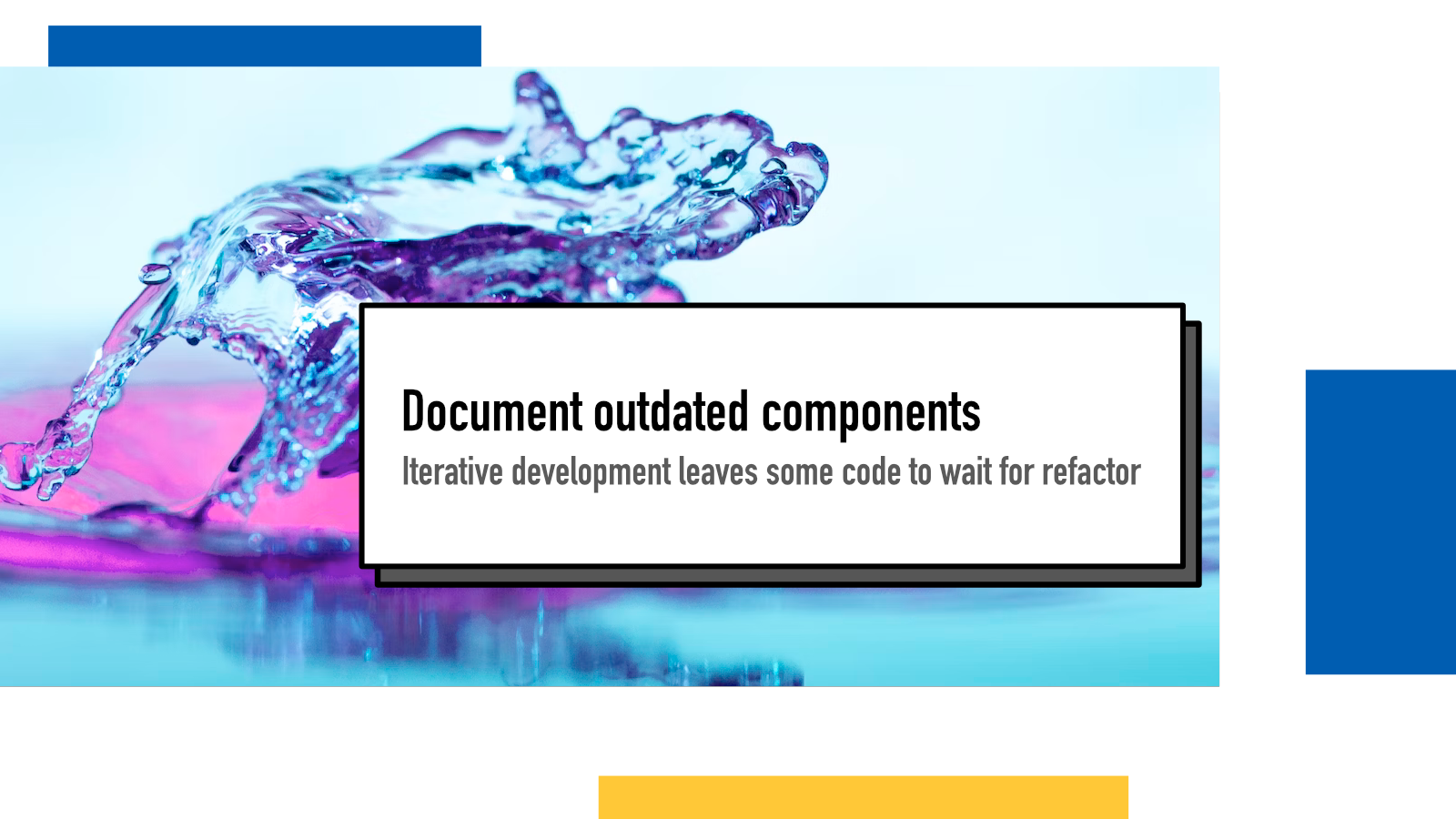
One of the realities of code bases that they don't teach you at school is that they evolve, often bit by bit. What that means is that there's rarely a "we decided to start doing one thing differently, let's rewrite everything to match that" moment – no matter how often we developers dream of being able to do that.
Document design via Storybook
Documenting visual elements and design is important to help maintain a consistent visual style and to make sure you use the right components at the right places when building your application.
This is especially important when there's no strong design oversight during the review process (for example, no designer in a team). I've seen it with my own eyes when an app ends up in a situation where there's 5 different <table>
styles across 7 different sub pages of the same tab group.
All of that because 1) the developer had to pick one and copy it as an example or design one from scratch and 2) because nobody caught it during review.
But if your team is just like so many other software teams in the world, you might not have that.
In a case like that, building and documenting a design system using something like Storybook is a great way. You could either mark the old components as deprecated or not document them at all, maybe marking them as something that should be brought up to speed with the rest whenever there's time to refactor.
Storybook has become one of my favorite tools lately as it allows you to document the visual and functional side of your application. With one look, without ever opening the code editor and trying to guess, you can find what kind of options you have for base components and more complex, specific components built on top of them. And you can tell the viewer what they mean, when they should use them and why they are like they are. That's brilliant, if you ask me.
You can document the different props given to a component and how they affect the visuals and function of a component. The developer can then go to their code editor and import the right component and pass the right props to it. And when creating new components, less guesswork and less opportunities to accidentally pick up an older deprecated one as a template.
Iterative improvements in coding style can lead astray
This also applies to coding style.
Let's say a team started working with React when class based components were all the recommended way to build components and they built a notification component for their news:
import React, { Component } from 'react
class NewsNotification extends Component {
render() {
<span class="notification-badge">{this.props.newsNotifications.length}</span>
}
}
function mapStateToProps(state) {
const { newsNotifications } = state
return { newsNotifications }
}
export default connect(mapStateToProps)(NewsNotification)
Now, let's say some years later the team shifted their development style to use function components and the next time they built a notification component for messages, they did it differently:
import React from 'react'
import { useSelector } from 'react-redux'
const MessageNotification = () => {
const messageNotifications = useSelector(state => state.messageNotifications)
return <span class="notification-badge">{messageNotifications.length}</span>
}
export default MessageNotification
Now, let's say the team hires a new developer and one of the early tasks is to create a notification component for a third type of notification in the system. A usual way they'll start looking into it is to look for something similar in the app, find it in the code and then use that as a blueprint.
Given that the system has two notification systems, it's roughly 50/50 which one they end up looking up as the example. Or they might build something that is bit more complex than our example based on that example and have 50/50 chance of picking the right one. Then, maybe in the code review, they hear the "oh, you should do it like this". Or worse, nobody picks it up during the review and the code base ends up diverting further and further apart.
It's common that these older files are refactored iteratively bit by bit – those of us who have been in projects that attempt large rewrites know that they very often lead to nothing getting finished because there's always something to fix.
In those cases, it's important to document this somewhere. Maybe in those files that your team has identified as future cases, you can add a comment that says
When you change this file the next time, refactor it to use [current style]
This has two benefits: first, it's less likely that this style gets copied to a new file and second, you are reminded to refactor it when you make other changes to the file.
If something above resonated with you, let's start a discussion about it! Email me at juhamattisantala at gmail dot com and share your thoughts. In 2025, I want to have more deeper discussions with people from around the world and I'd love if you'd be part of that.
Comments
Loading comments...
Continue discussion in Mastodon »