help() me Obi-Wan Kenobi, you’re my only hope
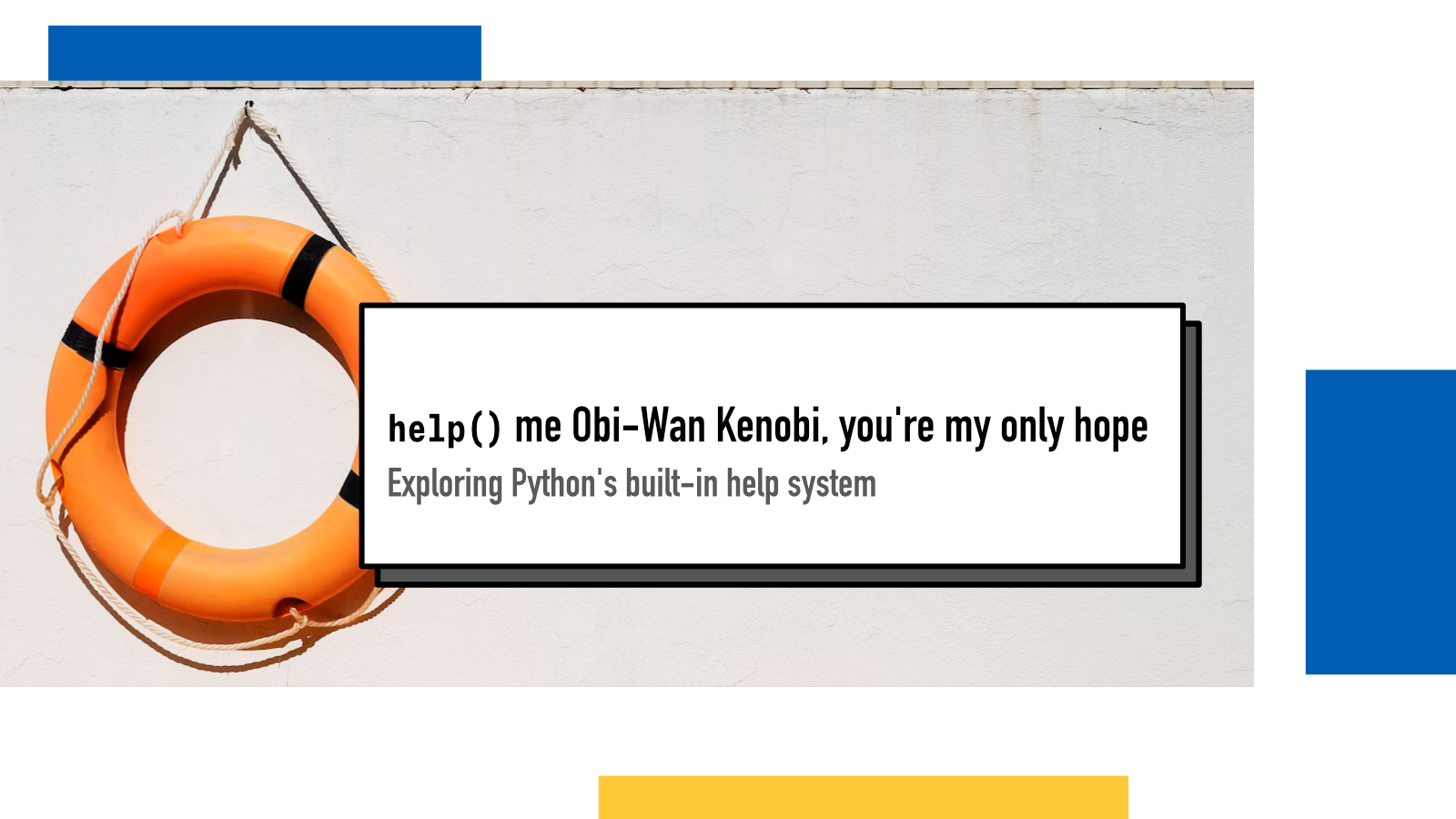
Batteries included is a blog series about the Python Standard Library. Each day, I share insights, ideas and examples for different parts of the library. Blaugust is an annual blogging festival in August where the goal is to write a blog post every day of the month.
Whether you are exploring Python, developing new features or debugging your code, the built-in help system is a great companion. It’s designed for interactive use, to be run in a REPL session.
Help for variables and objects
You can pass in almost anything to the help function and it will provide a
paged documentation for the given item. For example, asking help on
list
gives you the instructions on how
to create a list and all of its methods, their parameters and what they do:
>>> help(list)
Help on class list in module builtins:
class list(object)
| list(iterable=(), /)
|
| Built-in mutable sequence.
|
| If no argument is given, the constructor creates a new empty list.
| The argument must be an iterable if specified.
|
| Methods defined here:
|
| __add__(self, value, /)
| Return self+value.
|
| __contains__(self, key, /)
| Return key in self.
|
| __delitem__(self, key, /)
| Delete self[key].
|
| __eq__(self, value, /)
| Return self==value.
...
Alternatively, if you have a variable that you’re not quite sure what it is or you don’t remember what its methods are called, you can pass in a variable:
>>> items = dict()
>>> help(items)
Help on dict object:
class dict(object)
| dict() -> new empty dictionary
| dict(mapping) -> new dictionary initialized from a mapping object's
| (key, value) pairs
| dict(iterable) -> new dictionary initialized as if via:
| d = {}
| for k, v in iterable:
| d[k] = v
| dict(**kwargs) -> new dictionary initialized with the name=value pairs
| in the keyword argument list. For example: dict(one=1, two=2)
|
| Methods defined here:
|
| __contains__(self, key, /)
| True if the dictionary has the specified key, else False.
|
| __delitem__(self, key, /)
| Delete self[key].
|
| __eq__(self, value, /)
| Return self==value.
|
| __ge__(self, value, /)
| Return self>=value.
If you pass it a function or method, you’ll get the help for that specific function:
>>> from collections import namedtuple
>>> Point = namedtuple('Point', ['x', 'y'])
>>> origin = Point(0, 0)
>>> help(origin.count)
Help on built-in function count:
count(value, /) method of __main__.Point instance
Return number of occurrences of value.
Help on topics
If you invoke the help system without any arguments with
help()
, you’ll enter into a help
utility. Within it, you can explore modules, keywords, symbols and topics.
If you need help with Python’s various keywords, you can start a help session and type in “keywords”:
>>> help()
help> keywords
Here is a list of the Python keywords. Enter any keyword to get more help.
False class from or
None continue global pass
True def if raise
and del import return
as elif in try
assert else is while
async except lambda with
await finally nonlocal yield
break for not
help> break
The "break" statement
*********************
break_stmt ::= "break"
"break" may only occur syntactically nested in a "for" or "while"
loop, but not nested in a function or class definition within that
loop.
It terminates the nearest enclosing loop, skipping the optional "else"
clause if the loop has one.
If a "for" loop is terminated by "break", the loop control target
keeps its current value.
When "break" passes control out of a "try" statement with a "finally"
clause, that "finally" clause is executed before really leaving the
loop.
Related help topics: while, for
Within the modules help section, you can find documentation on Python’s standard library packages.
>>> help()
help> collections
Help on package collections:
NAME
collections
MODULE REFERENCE
https://docs.python.org/3.10/library/collections.html
The following documentation is automatically generated from the Python
source files. It may be incomplete, incorrect or include features that
are considered implementation detail and may vary between Python
implementations. When in doubt, consult the module reference at the
location listed above.
DESCRIPTION
This module implements specialized container datatypes providing
alternatives to Python's general purpose built-in containers, dict,
list, set, and tuple.
* namedtuple factory function for creating tuple subclasses with named fields
* deque list-like container with fast appends and pops on either end
* ChainMap dict-like class for creating a single view of multiple mappings
* Counter dict subclass for counting hashable objects
* OrderedDict dict subclass that remembers the order entries were added
* defaultdict dict subclass that calls a factory function to supply missing values
* UserDict wrapper around dictionary objects for easier dict subclassing
* UserList wrapper around list objects for easier list subclassing
* UserString wrapper around string objects for easier string subclassing
...
I learned the existence of this utility from Ishaan’s great blog post Getting help [in Python] earlier this year.
A sidenote on dir()
Another helpful tool that I use during interactive sessions is dir() that returns a list of valid attributes for a given object. I often use it to check what were the names of attributes and methods a given variable has:
>>> a = dict()
>>> dir(a)
['__class__', '__class_getitem__', '__contains__', '__delattr__',
'__delitem__', '__dir__', '__doc__', '__eq__', '__format__',
'__ge__', '__getattribute__', '__getitem__', '__getstate__',
'__gt__', '__hash__', '__init__', '__init_subclass__', '__ior__',
'__iter__', '__le__', '__len__', '__lt__', '__ne__', '__new__',
'__or__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__',
'__ror__', '__setattr__', '__setitem__', '__sizeof__', '__str__',
'__subclasshook__', 'clear', 'copy', 'fromkeys', 'get', 'items',
'keys', 'pop', 'popitem', 'setdefault', 'update', 'values']
Sometimes I find dir()
faster and easier
to find things at glance, especially when I know what I’m looking for but am
not exactly sure what it’s called or how its spelled, than opening the full,
multi-page help manual help()
offers.
Whenever I need to know more, like the arguments provided or need to figure
out the name of a method based on descriptions, I reach for
help()
.
If something above resonated with you, let's start a discussion about it! Email me at juhamattisantala at gmail dot com and share your thoughts. In 2025, I want to have more deeper discussions with people from around the world and I'd love if you'd be part of that.