map and filter with list comprehensions
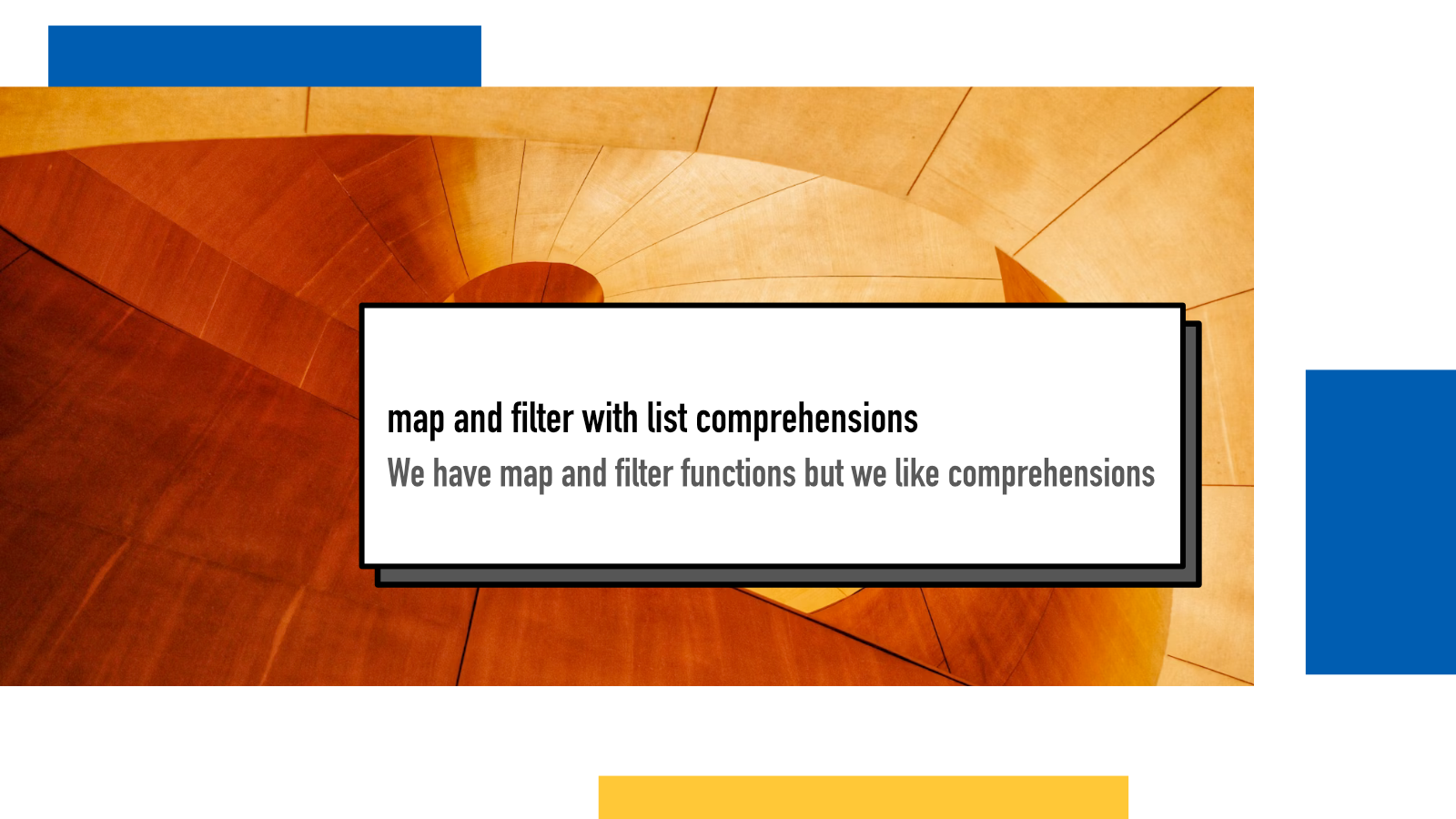
Batteries included is a blog series about the Python Standard Library. Each day, I share insights, ideas and examples for different parts of the library. Blaugust is an annual blogging festival in August where the goal is to write a blog post every day of the month.
In functional programming, there are two key operations that are used with iterables: map and filter.
Map runs a function on each item of an iterable and filter runs a predicate on
each item and only returns the ones that results in truthy value. In Python,
there are built-in functions
map
and
filter
but they are not used that much because Python has something even better: list
comprehensions.
What’s a list comprehension?
A list comprehension is a construct that allows creation of lists with a handy syntax:
# Basic syntax
ns = [n for n in iterable]
# same as
ns = []
for n in iterable:
ns.append(n)
The basic example doesn’t do much because it doesn’t manipulate the input in any way.
pairs = [(1, 'a'), (2, 'b'), (3, 'c')]
numbers = [num for num, _ in pairs]
# = [1, 2, 3]
In this second example, we extract only the first item of each pair into a new list.
Map and filter
Let’s see how we can use map
:
numbers = [1,2,3,4,5,6]
def square(n):
return n*n
squares = list(map(square, numbers))
# squares = [1, 4, 9, 16, 25, 36]
squares = [num * num for num in numbers]
# squares = [1, 4, 9, 16, 25, 36]
and filter:
numbers = [1,2,3,4,5,6]
def is_odd(n):
return n % 2 != 0
odds = list(filter(is_odd, numbers))
# odds = [1, 3, 5]
odds = [num for num in numbers if num % 2 != 0]
# odds = [1, 3, 5]
For those who come from a different language, this can feel a bit weird and unnecessary but it’s a rather beloved syntax for pythonistas to deal with lists.
What I like about it especially is how smoothly it reads as English.
If something above resonated with you, let's start a discussion about it! Email me at juhamattisantala at gmail dot com and share your thoughts. In 2025, I want to have more deeper discussions with people from around the world and I'd love if you'd be part of that.