Random number chosen by fair dice roll
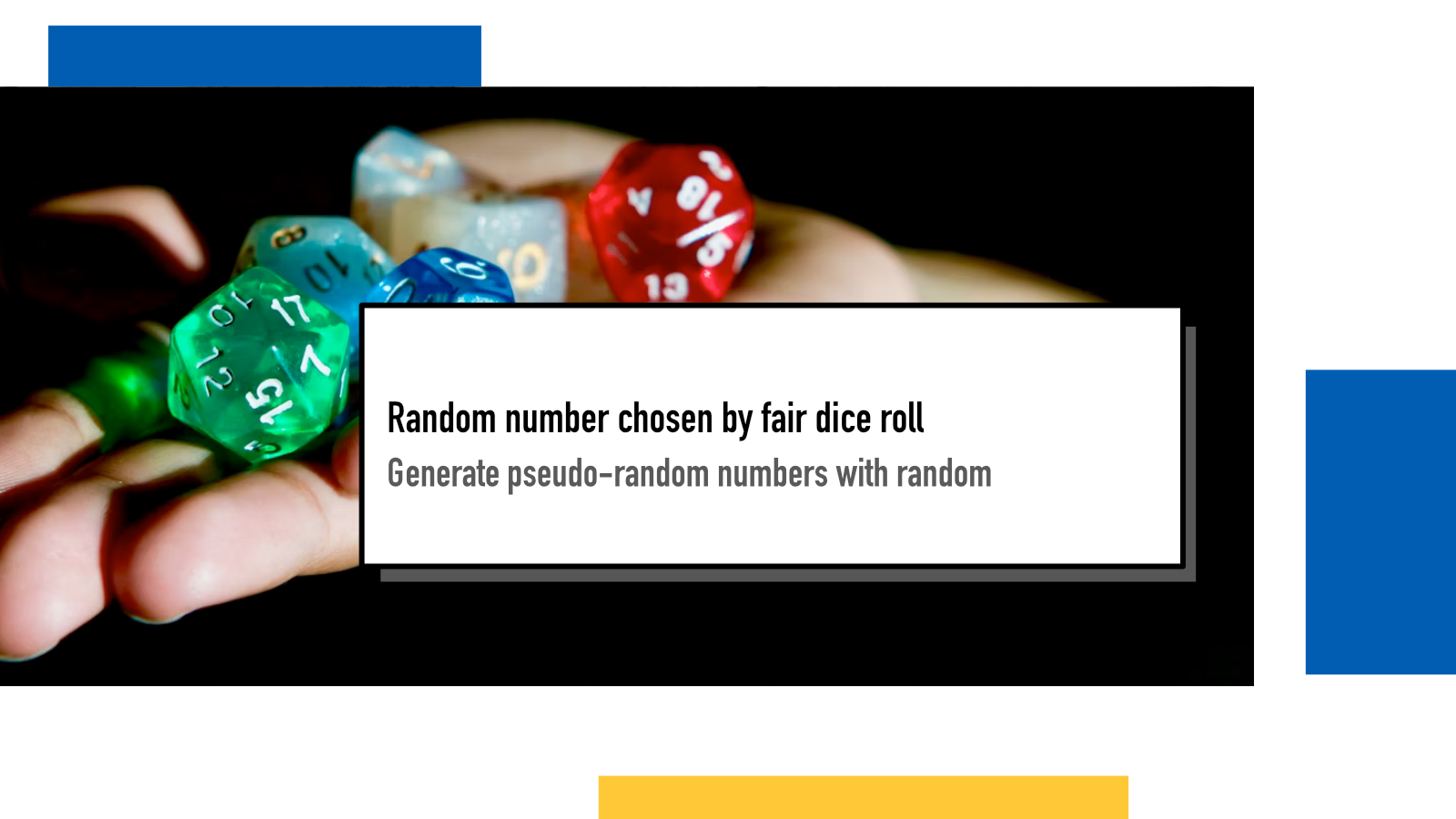
Batteries included is a blog series about the Python Standard Library. Each day, I share insights, ideas and examples for different parts of the library. Blaugust is an annual blogging festival in August where the goal is to write a blog post every day of the month.
Most of the time we want the program do what we tell it to do. (and when they don’t, Syntax Error newsletter exists to help you)
However, sometimes a bit of surprise is nice and that’s when we tell the computer to give us something specific randomly. Python’s random module offers tooling to generate pseudo-random numbers.
A note from the documentation:
Warning
The pseudo-random generators of this module should not be used for security purposes. For security or cryptographic uses, see the secrets module.
Simulating dice
Random module is handy if we’re for example simulating a die:
import random
def roll_d6():
# returns a random int between 1 and 6
return random.randint(1, 6)
def roll_d20():
# for the DnD players reading
return random.randint(1, 20)
print(roll_d6())
print(roll_d6())
print(roll_d20())
Random selection
When you want to pick one or more random items from a sequence like list,
random
offers a few methods for that:
import random
vowels = ['a', 'e', 'i', 'o', 'u']
# Returns one of the 5 vowels
random.choice(vowels)
# Select 3 vowels (repeats are allowed)
random.choices(vowels, k=3)
# Define different weights (probabilities)
random.choices(vowels, weights=[40, 15, 15, 15, 15], k=3)
# Select 3 vowels (no repeats)
random.sample(vowels, k=3)
Defining determinism
Randomness can make testing the program difficult because the outcome can
always be different from one run to another. When using
random
, you can define
a seed
which makes all the subsequent random rolls deterministic:
import random
random.seed(101010)
print(random.randint(1, 10)) # 8
print(random.randint(1, 10)) # 1
print(random.randint(1, 10)) # 2
print(random.randint(1, 10)) # 6
This way, you can use randomness in your application but set the seed at the beginning of tests and then test your other logic knowing the results of random values..
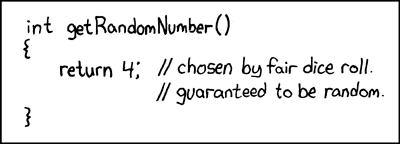
The title of the post is a homage to the classic xkcd comic Random Number. Image shared in accordance with the Creative Commons Attribution-NonCommercial 2.5 License.
If something above resonated with you, let's start a discussion about it! Email me at juhamattisantala at gmail dot com and share your thoughts. In 2025, I want to have more deeper discussions with people from around the world and I'd love if you'd be part of that.