Test your codebase with unittest
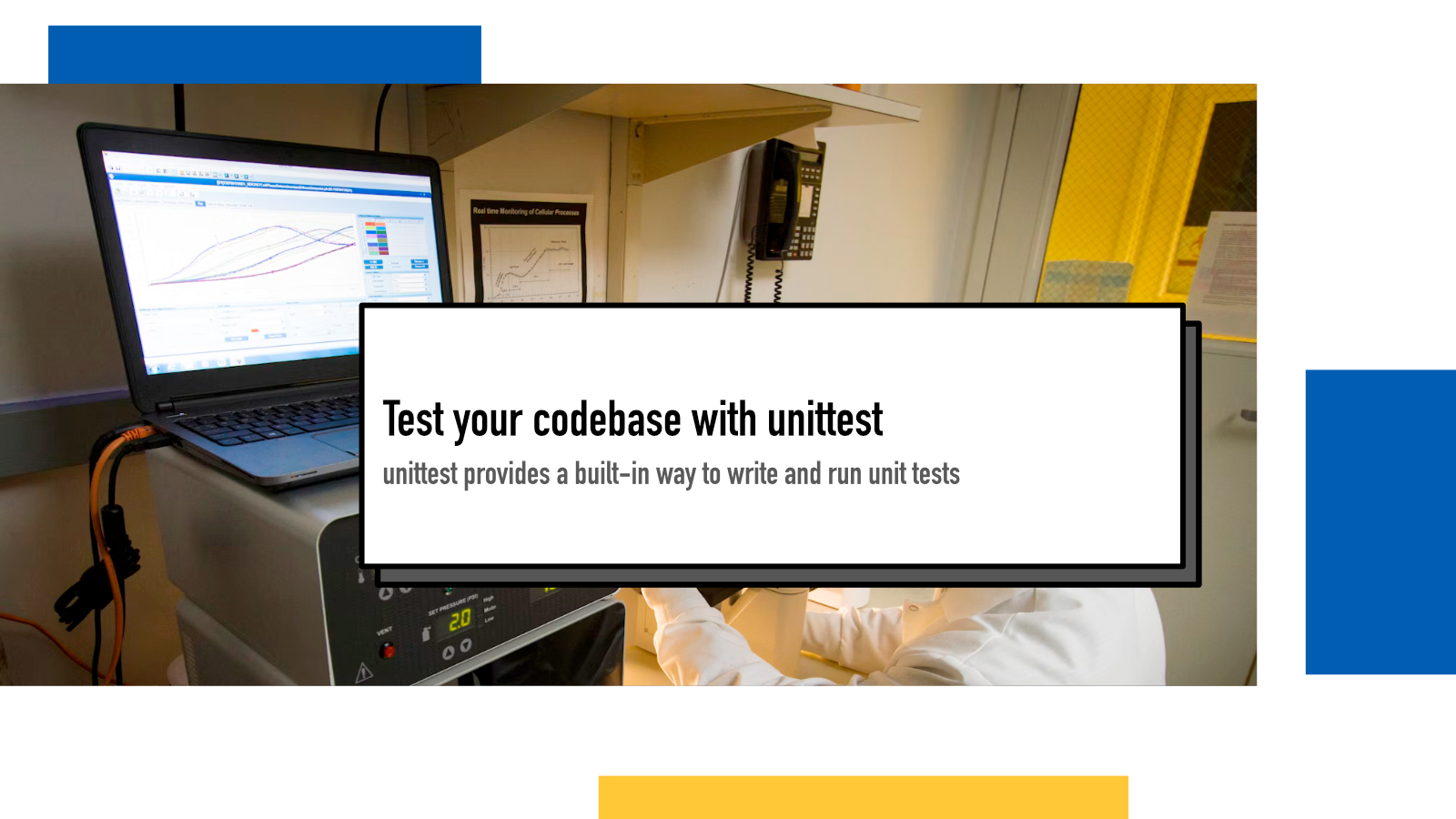
Batteries included is a blog series about the Python Standard Library. Each day, I share insights, ideas and examples for different parts of the library. Blaugust is an annual blogging festival in August where the goal is to write a blog post every day of the month.
Python provides a couple of ways to write automated tests for your code, built in to the standard library. On day 8th of Batteries included, I wrote about how you can use doctest to not only test but also document your code. Doctest does have its disadvantages though so here’s another option: using unittest.
Basics of using unittest
To start using unittest, there are a couple of things you need to do:
- Import unittest module
-
Write your tests in a class that inherits from
unittest.TestCase
-
Run
unittest.main()
In a real test, you would also import your own modules that you’re testing into the file.
You can add multiple test methods to the class and it’s up to you how to divide tests to different classes.
Each test needs to start with a test_
to
be included. If you have code that needs to run before or after each test, you
can add setUp
and
tearDown
methods respectively.
TestCase class comes with a selection of assertion methods for you to use to compare values.
Document further with optional messages
You can add an optional third parameter to these assertion methods as a custom message that gets printed if the test fails. I find this very handy for documenting the why of a test in a way that can be challenging to fit into a method name:
self.assertEqual(len(create_list_of_four()), 4, 'List generator should always return a list length of four')
AssertionError: 3 != 4 : List generator should always return a list length of four
Method names help identify the test in the code but I always appreciate if I can find out what issues are being caused by the failing tests as that usually helps me identify the possible causes and help debug the issues further.
If something above resonated with you, let's start a discussion about it! Email me at juhamattisantala at gmail dot com and share your thoughts. In 2025, I want to have more deeper discussions with people from around the world and I'd love if you'd be part of that.