The ability to code sometimes feels like magic to me
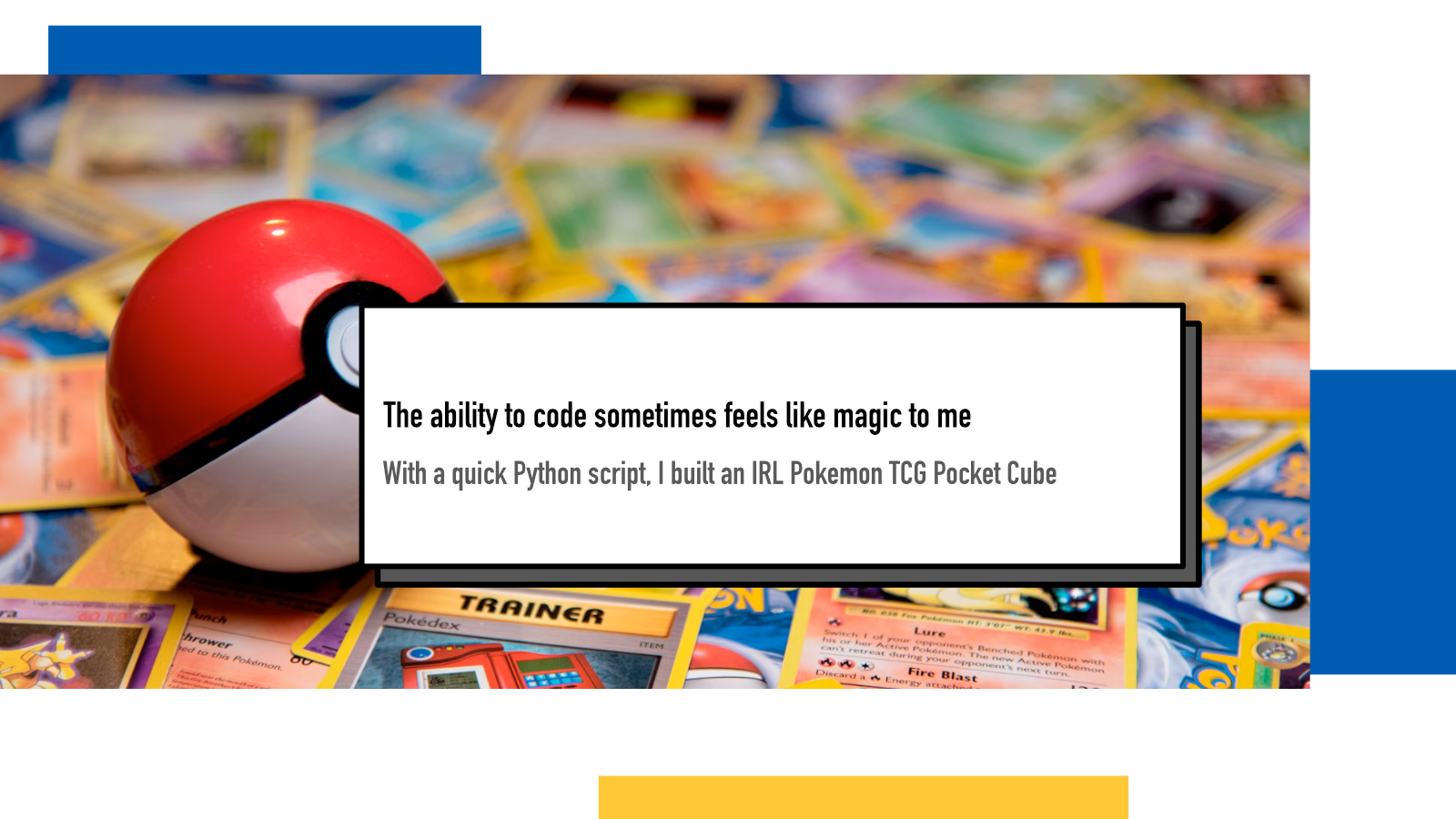
Have you ever read books or watched series or movies with magic and wizardry and thought to yourself: “It would be cool if I could swing my wand or mumble some ancient incantations and things would happen”?
I have and the closest I’ve got to that feeling is writing small programs for myself.
Last summer, for IndieWeb Carnival I wrote about the concept of home-cooked, situated software and just yesterday I was reading Robert Alexander’s Designing Personal Software and we both came to a conclusion that it’s awesome to build stuff for your own needs.
While I was browsing the web today, I came across SWU CUBES’ Youtube video about a 2-player Pokemon TCG Pocket cube that he had designed. I love cubes, especially small 2-player ones that fit into a small 80 or 100 card deck boxes. He was kind enough to provide the cube list as a Google spreadsheet and I decided to build the cube for myself too. I’m not a big fan of Pocket’s mobile experience and it’s “you need to open the app every N hours to advance” paradigm but I think the format can be a great way to teach someone new to the game or play some casual games while sipping a pint in a pub.
There are many ways one could take to turn the spreadsheet into a playable paper cube. One could look at each card, search for its picture, copy and paste it into a Word document, adjust sizes and print them out. Then cut the cards out, sleeve them with a bulk card in the back and a few laborious hours later, you’d have a cube to play with.
I decided to take another approach. I wrote a custom Python script that did most of that for me.
The process
Step 1: The script
The first part of the process goes like this:
- Download the spreadsheet as HTML
-
Find all cards based on their
[set] [number]
pattern - For each card, download the image from a site that has Pocket images
"""
Read cube list from a Google Sheet and download the corresponding card images.
"""
import re
import requests
from pathlib import Path
# Cube list spreadsheet info
DOCS_KEY = "10F7JE__svhLJ3ANHw_KZQoAGVNRR1Xkhl0ncnmWD75Y"
SHEET_NAME = "Small Cube"
FORMAT = "html"
SPREADSHEET_URL = f"https://docs.google.com/spreadsheets/d/{DOCS_KEY}/gviz/tq?tqx=out:{FORMAT}&sheet={SHEET_NAME}"
# How the cards are defined
CARD_PATTERN = r"(A1a?|P-A) (\d{2,3})"
# Where to get the images
CARD_IMAGE_URL = "https://static.dotgg.gg/pokepocket/card/"
def get_html(url):
response = requests.get(url)
return str(response.content)
def download_image(url, save_as):
response = requests.get(url)
with open(save_as, "wb") as file:
file.write(response.content)
if __name__ == "__main__":
html = get_html(SPREADSHEET_URL)
cards = re.findall(CARD_PATTERN, html)
Path("cards").mkdir(parents=True, exist_ok=True)
for set, number in cards:
if set == "A1a":
number = f"0{number}"
elif set == "P-A":
set = "PROMO"
filename = f"{set}-{number}.webp"
download_image(f"{CARD_IMAGE_URL}{filename}", f"cards/{filename}")
print(f"Downloaded {len(cards)} images")
There are three types of card ids in this cube:
-
A1 219
: a set A1 with a three digit number for the card -
A1a 01
: a set A1a with a two digit number for the card -
P-A 001
: a set P-A with a three digit number for the card
-
(A1a?|P-A)
means: find and capture either -
(\d{2,3})
means: find and capture a number with 2 or 3 digits
Due to how the site I used to download the images from has slightly different naming scheme, I have to do two adjustments:
- If the set is “A1a”, we need to add an extra 0 to make the card number a three digit one
- If the set is “P-A”, it needs to be “PROMO” instead
Step 2: Printing the proxies
I have been building and prototyping all sorts of card games for years and needed a quick way to print card-sized prints that can be cut and sleeved with bulk cards for playtesting.
That small tool lives in https://hamatti.org/proxy where I can drag-and-drop any images that fit the ratio of a 2.5” x 3.5” card and I use my own card-print.css styling library to then print the cards in a way that 9 cards fit an A4 paper and they are just slightly smaller than real cards, making them easier to cut and sleeve.
Some of the cards in the cube have a quantity higher than 1 so I drag and drop them multiple times. If there were significantly more of them, I’d take that into account in my script but here it was only a couple so it was easier to do manually.
The cube also includes 2 Poke Ball and 2 Professor Research cards that are given to both players so I manually added them to my prints.
Aftermath
The only thing then left to do is to hit the printer, buy new sleeves and a deckbox for the cube from the local game store and start playing.
I don’t know if I saved time compared to doing it manually but I sure did save my sanity. And I had a lot of fun. I can’t wait to bring this small cube to my gaming group and test it out.
I don’t think the author talked about how his planning this cube to be drafted but I’m planning to use Solomon Cube as explained by Andrew Mahone in his video. There are 81 cards in the cube so the last card will be undrafted. Players then craft 20 card decks and have a set of energies that they can take one per turn from the energy zone.
If something above resonated with you, let's start a discussion about it! Email me at juhamattisantala at gmail dot com and share your thoughts. In 2025, I want to have more deeper discussions with people from around the world and I'd love if you'd be part of that.