Added keyboard support to ON24 with bookmarklet
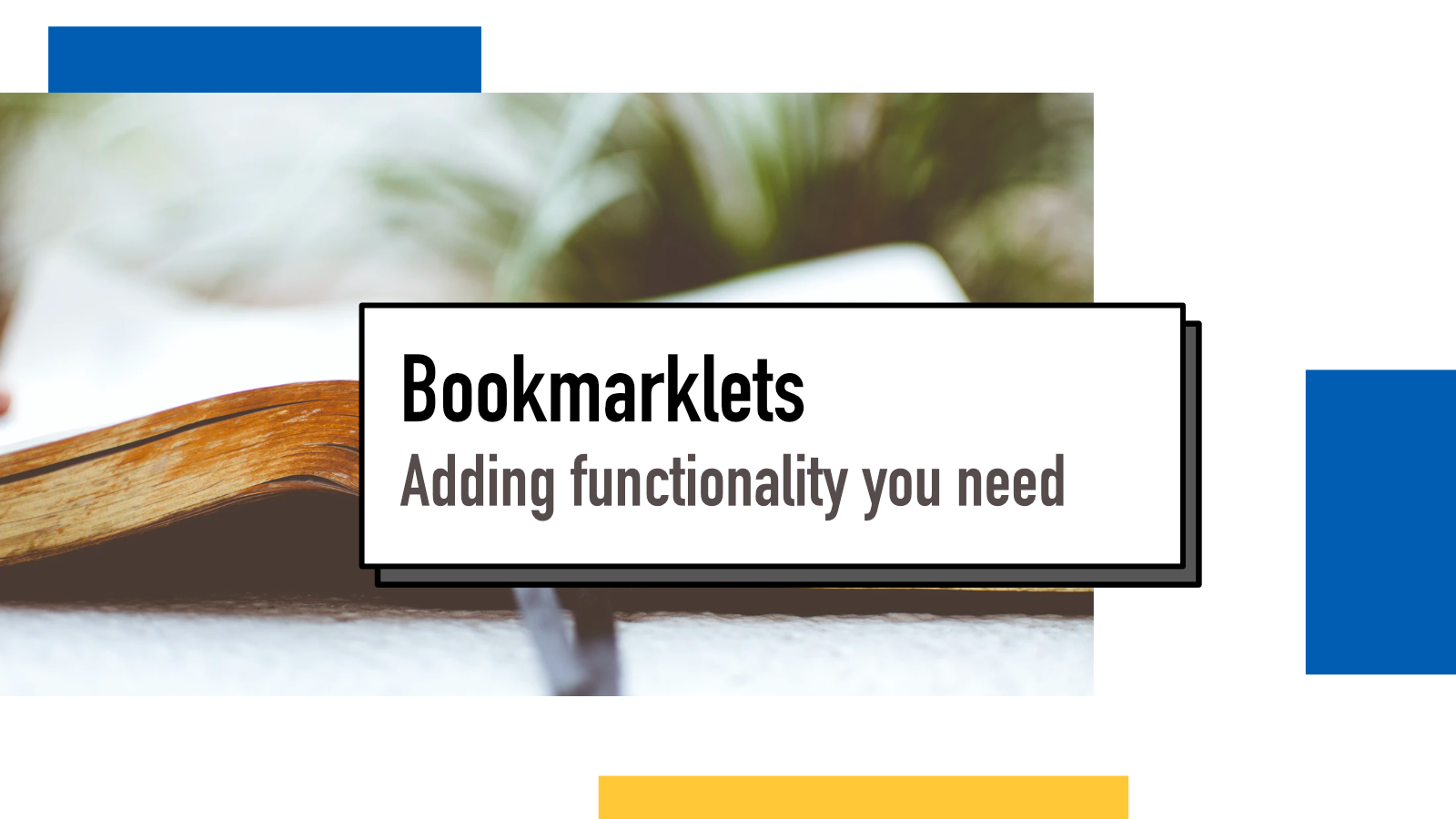
Bookmarklets are fantastic. Not having a keyboard support for a web app is less fantastic. Here's a short story and bookmarklet of how I added keyboard (and by association, presentation clicker) support to ON24's Webinar platform.
Two weeks ago I was preparing to give a talk at sthml.js meetup remotely from my home studio (I call it a studio: it's a webcam on tripod + wireless mic) and we were using ON24 as a webinar platform for that meetup.
During our rehearsal session the day before the meetup, I noticed that there was no keyboard support for switching slides which also meant that my Targus presentation clicker (that emulates(?) keyboard) didn't work either. I had to move my mouse over a small arrow and click that – not a fun thing to do when I'm standing in front of a camera and talking to an audience across the pond.
As someone who likes to hack things to build the functionality I need, I figured I can do this by myself. Instead of building a Chrome extension for this that I knew I'd maybe need that one time instead of constantly, I went to the bookmarklet route.
Bookmarklets are small Javascript scripts that are triggered when a user clicks on a bookmark that contains that code. Since all I needed here was to translate some key presses into Javascript click events, I figured bookmarklet is the simplest way.
Here's the full code. I started by adding functionality for left/right arrow keys but then learned that my clicker actually sends PageUp and PageDown presses so I added them in.
const prev = document.querySelector('.arrow-btn.prev')
const next = document.querySelector('.arrow-btn.next')
const LEFT_ARROW = "ArrowLeft"
const RIGHT_ARROW = "ArrowRight"
const PAGE_DOWN = 'PageDown'
const PAGE_UP = 'PageUp'
document.onkeyup = event => {
if(event.code === LEFT_ARROW || event.code === PAGE_UP) {
prev.click();
} else if (event.code === RIGHT_ARROW || event.code === PAGE_DOWN) {
next.click();
}
}
In bookmarklet, it looks like this:
javascript:void(function() { const prev = document.querySelector('.arrow-btn.prev'); const next = document.querySelector('.arrow-btn.next'); const LEFT_ARROW = "ArrowLeft"; const RIGHT_ARROW = "ArrowRight"; const PAGE_DOWN = 'PageDown'; const PAGE_UP = 'PageUp'; document.onkeyup = event => { if(event.code === LEFT_ARROW || event.code === PAGE_UP) { prev.click(); } else if (event.code === RIGHT_ARROW || event.code === PAGE_DOWN) { next.click(); } }})()
To create a bookmarklet, you can drag ON24 KEYBOARD SUPPORT to your bookmark bar. Then you navigate to your presenter mode in ON24 and click the bookmarklet.
Voilà, you have keyboard and clicker support.
If something above resonated with you, let's start a discussion about it! Email me at juhamattisantala at gmail dot com and share your thoughts. In 2025, I want to have more deeper discussions with people from around the world and I'd love if you'd be part of that.