Automate filling a form with a bookmarklet
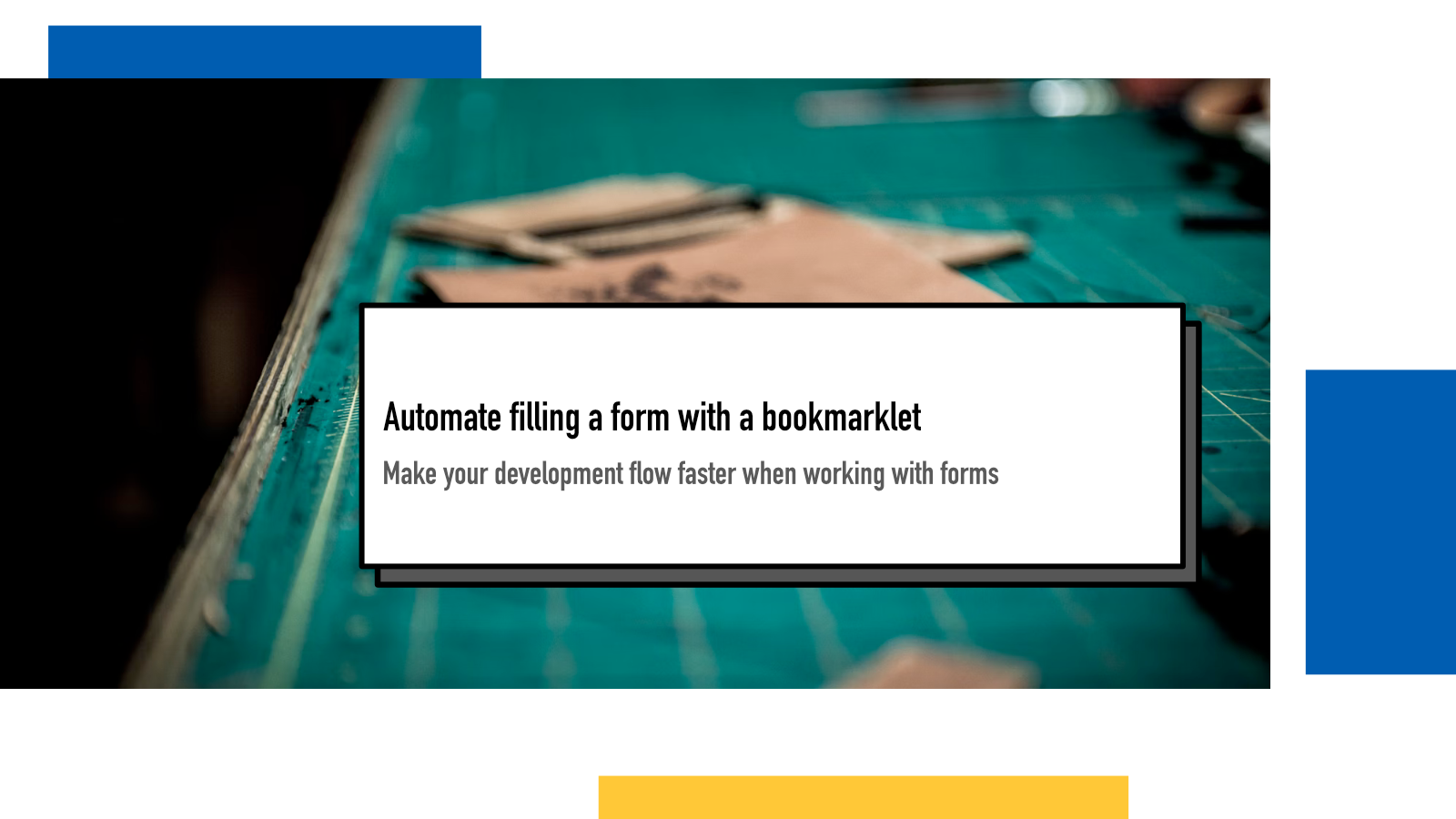
Web developers often need to fill in forms over and over again as we develop websites and applications that are built around those forms. Not only is it boring but that boredom can lead to us entering silly or funny entries that can sometimes accidentally leak during demos or screenshots or database dumps and lead to negative outcomes.
That’s where bookmarklets come in handy!
Bookmarklets are handy scripts that run Javascript and you can make Javascript do the work for you to keep filling those forms.
With a custom bookmarklet, you click it once and let it fill the form, allowing you to focus on the important development work rather than spending your working hours filling in the same form with dummy content over and over.
Select an element
First step is to figure out how you’re gonna find and select the items in your
form. Using
document.querySelector
combined with
CSS selectors
offers you a wide range of options.
Since we’re building a bookmarklet for our own use, we don’t have to make it resistant to changes in the code as we always have the possibility to change it if the underlying HTML changes.
Here’s an example form with a couple of different inputs:
And as code:
<form aria-labelledby="form-heading">
<h3 id="form-heading">Example form</h3>
<div
style="
display: grid;
grid-template-columns: auto auto;
width: 500px;
justify-items: start;
gap: 0.5em;
"
>
<label for="name">Name:</label> <input type="text" id="name" />
<label for="agree">Agree?</label> <input type="checkbox" id="agree" />
<label for="select">Ice cream flavour</label>
<select id="select">
<option value="">--Pick your favorite flavour--</option>
<option value="1">Vanilla</option>
<option value="2">Chocolate</option>
<option value="3">Strawberry</option>
</select>
<label for="date">Date</label>
<input type="date" id="date" />
</div>
</form>
We can select our inputs with
document.querySelector('#[id-here]')
where we replace [id-here]
with the
corresponding id.
It’s possible that sometimes you don’t have ids set for every input. In that case, you need to find a CSS selector that matches your input.
For example, some may use
data-testid
for testing purposes which
is handy for bookmarklets too. In that case, you’d use
document.querySelector('[data-testid="[id-here]"]')
.
Or you might not have any in which case you can use
:nth-of-type
pseudoclass:
document.querySelector(’input:nth-of-type(2)')
would select our Agree? checkbox as it’s the second
input
element on the page.
Learning about the different possible CSS selectors is a good way to become more efficient as a frontend developer.
Set values
For an input
element, you can set a
value through value
property:
document.querySelector('#name').value = 'Juhis';
Checkboxes use a checked
property:
document.querySelector('#agree').checked = true;
For dropdown select
s, you set the value
to the desired underlying value of the
option
you want to select:
// Selects chocolate because it's value is 2
document.querySelector('#select').value = 2;
Elements like the date picker normalize the value into
YYYY-MM-DD
format so you need to pass in
the desired date in that format:
document.querySelector('#date').value = '2024-09-26';
Utilize Dev Tools for quick feedback loop
Browsers come with great developer tools that can help you experiment and find the right selectors and code for changing the values. I use it all the time when I’m creating bookmarklets.
I open the dev tools console and run selectors to make sure they pick up the correct elements and double check that my code to change values is correct.
This helps make sure that my code works before I make it into a bookmarklet. Going step-by-step makes debugging way easier compared to debugging a full bookmarklet.
Into a bookmarklet
Once you have all of your selectors and value assignments done, Caio’s Bookmarklet Maker is a fantastic tool to turn your readable Javascript code into a bookmarklet that you can drag and drop to your bookmarks bar.
You can test mine by dragging the link below to your bookmarks bar, clicking it and seeing how it fills the above form with set values.
If something above resonated with you, let's start a discussion about it! Email me at juhamattisantala at gmail dot com and share your thoughts. In 2025, I want to have more deeper discussions with people from around the world and I'd love if you'd be part of that.