Functions 101
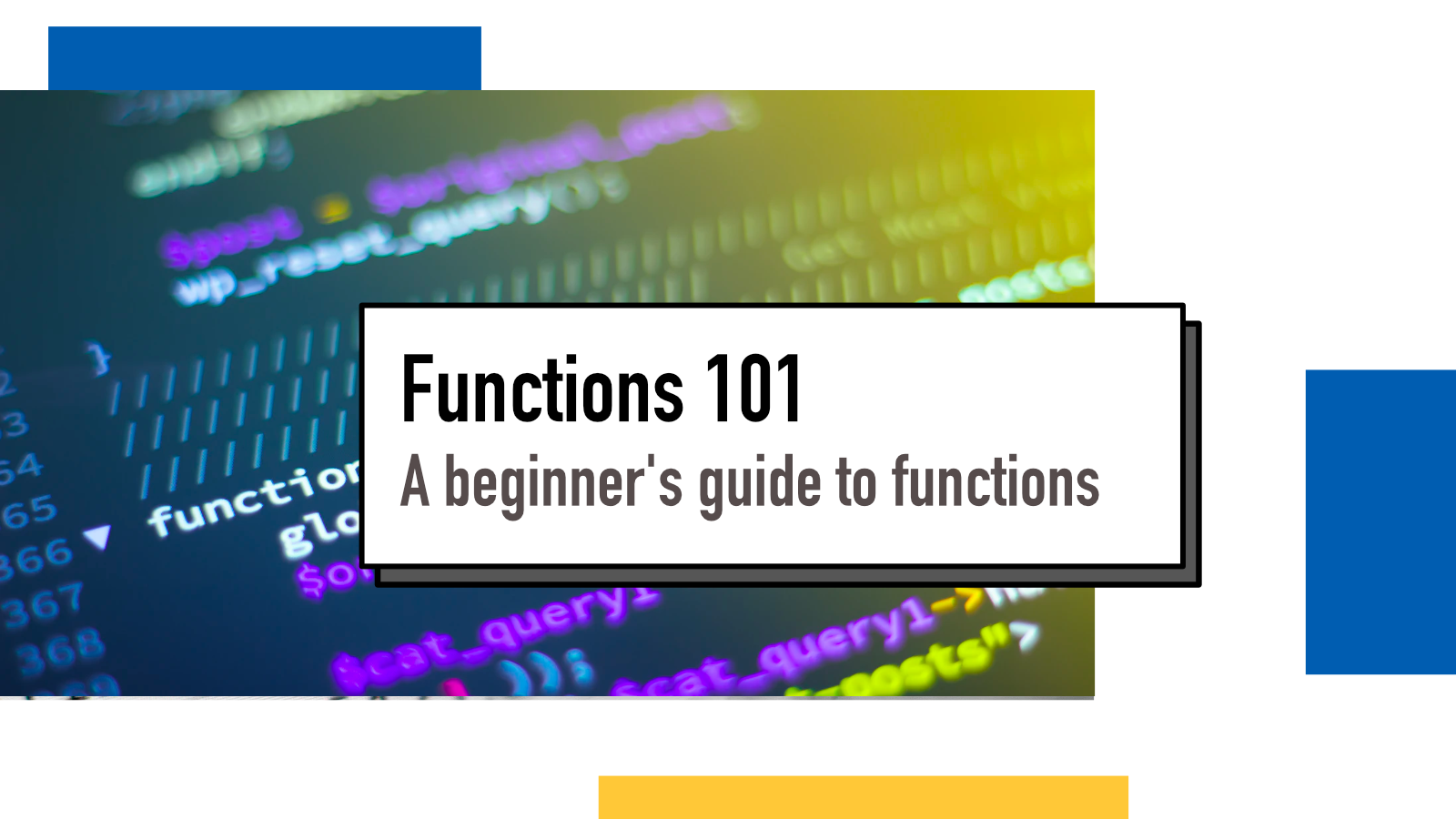
This blog post is aimed for beginners. If you have already built some software, it's very likely you won't get much out of it but you're welcome to stay for the ride. Just so you know.
There is a brief moment in new software developer's life when the concept of functions (or subroutines or methods as they are called in some languages) is bit fuzzy. This post aims to be a good starting point on your journey to understand functions, how they are built and how they are used.
I will be using Javascript as an example language but most of this applies to other languages as well.
Anatomy of a function
Let's start by looking at an example function:
function calculateAverage(x, y, z) {
const sum = x + y + z;
const average = sum / 3.0;
return average;
}
Here we have a function called calculateAverage
that calculates the average of three numbers.
calculateAverage
is the name of the function and it takes three parameters or arguments (called x
, y
and z
).
The name of the function as well as the names of the parameters are up to you as a developer to decide when creating a function. However, it's not completely arbitrary what you name them as they should be named to be as descriptive as possible for people reading it. For the computer, it doesn't matter if your function is called abc
or calculateAverage
but for humans reading your code and calling the function, it does.
A function can have 0, 1 or multiple parameters.
Inside {
and }
is what is called the body of the function. It's the code that gets executed when the function is being called (well get to that later). The body can contain multiple lines and it can call other functions inside it.
Optionally, a function can return a value. In Javascript (like many other languages), it's done by using the keyword return
followed by the value being returned. We'll see bit later what it means to return a value.
Using functions
There are three steps when using functions and this is something that I often see beginners being very confused with, so let's walk through step by step:
Defining a function
function calculateAverage(x, y, z) {
const sum = x + y + z;
const average = sum / 3.0;
return average;
}
We start by defining a function. This piece of code doesn't effectively do anything yet. It just creates a function into our software that we can use later in other parts. For example, if you find a function like this from a tutorial or as an answer to a question, simply copy-pasting it into your codebase doesn't do anything on its own.
Some functions are defined by the coder themselves, some come from libraries (pieces of code other developers have written and shared so you can use them) and some from the standard library of the language (meaning functions that are built-in to the programming language of your choice). All of them function similarly.
Calling a function
A function is called by writing its name and passing any parameters inside parentheses.
calculateAverage(1, 7, 25)
With this line of code, we tell our software to run the contents of the function with values 1
, 7
and 25
as parameters. What happens then is that the computer will run the function, calculate the average and return it. However, after that, the computer simply discards the return value and continues like nothing happened.
A side step: what is a scope?
To go deeper into this issue, we need to talk briefly about scope. Scope in plain English is the visibility area of a variable or function. In practice, it means where a variable or function is available to other pieces of code to run.
Let me demonstrate this with an example:
const number = 42;
function calculateAverage(x, y, z) {
const sum = x + y + z;
const average = sum / 3.0;
return average;
}
calculateAverage(1, 7, 25);
console.log(average);
Here we have one function (calculateAverage
), three variables (number
, sum
and average
) and three parameters (x
, y
and z
). They have different scopes, i.e. they can be read in different places.
Here, number
is available everywhere. It's defined on the top-level and it's scope is this entire script. Function calculateAverage
has the same scope as number
since they are defined at the same level.
Parameters x
, y
and z
and variables sum
and average
are only available inside the {
and }
– or as well learned earlier: body of the function. This means that even though the code ran and calculated the average of those three numbers into a variable average
, that is not available at the last line. Instead, you'll get an error ReferenceError: average is not defined
.
Understanding the scope is crucial for a software developer. It's not the easiest though since different programming languages define scope differently so you need to learn it for each one separately. But once you grasp it, it'll stay with you for the rest of your coding career.
Capturing the value
Third step of calling a function is to capture the value into a variable.
function calculateAverage(x, y, z) {
const sum = x + y + z;
const average = sum / 3.0;
return average;
}
const average = calculateAverage(1, 7, 25);
console.log(average);
On line 8 in the above code, we changed it to const average = calculateAverage(1, 7, 25);
and now the result of our function call is stored in a local variable called average
.
Please note that this average
is different from the average
on the line 5. You cannot have the two variables with the same name in the same scope but here, line 5 average
only exists within that scope.
We could call the average
on line 8 anything. It's not tied in any way to the average
we calculated in line 5.
For us to be able to capture these values, the function needs a return
statement. If we don't have a return
, a function always returns undefined
in Javascript. However in some other languages, they might return other things: Python returns None
and Ruby actually doesn't need to have return
at all, it will always return the last expression of the function.
Common mistakes
Some common mistakes I see beginner programmers do are:
- Not realizing a difference between defining a function and calling a function. Just defining it with
function hello()
does not run it, you need to explicitly call it. - Not understanding the scope of variables and then trying to access variables created inside a function scope rather than returning the desired value.
- Not remembering to store the output of a function into a variable.
A word of encouragement if you're in this phase as a developer: it will pass. This is something you need to learn once and after that, you got it.
Wrap up
First you define a function by telling the computer what this function will do. Then you call it to actually run it with real values. Finally, you capture the output into a variable so it's not lost.
There are other advanced things you can do with functions but they are not important right now. I have left them out for now so you won't get confused.
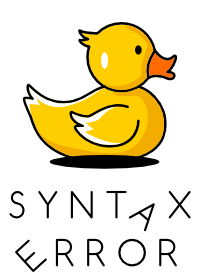
Sign up for Syntax Error, a monthly newsletter that helps developers turn a stressful debugging situation into a joyful exploration.