I wish Python had Integer.times
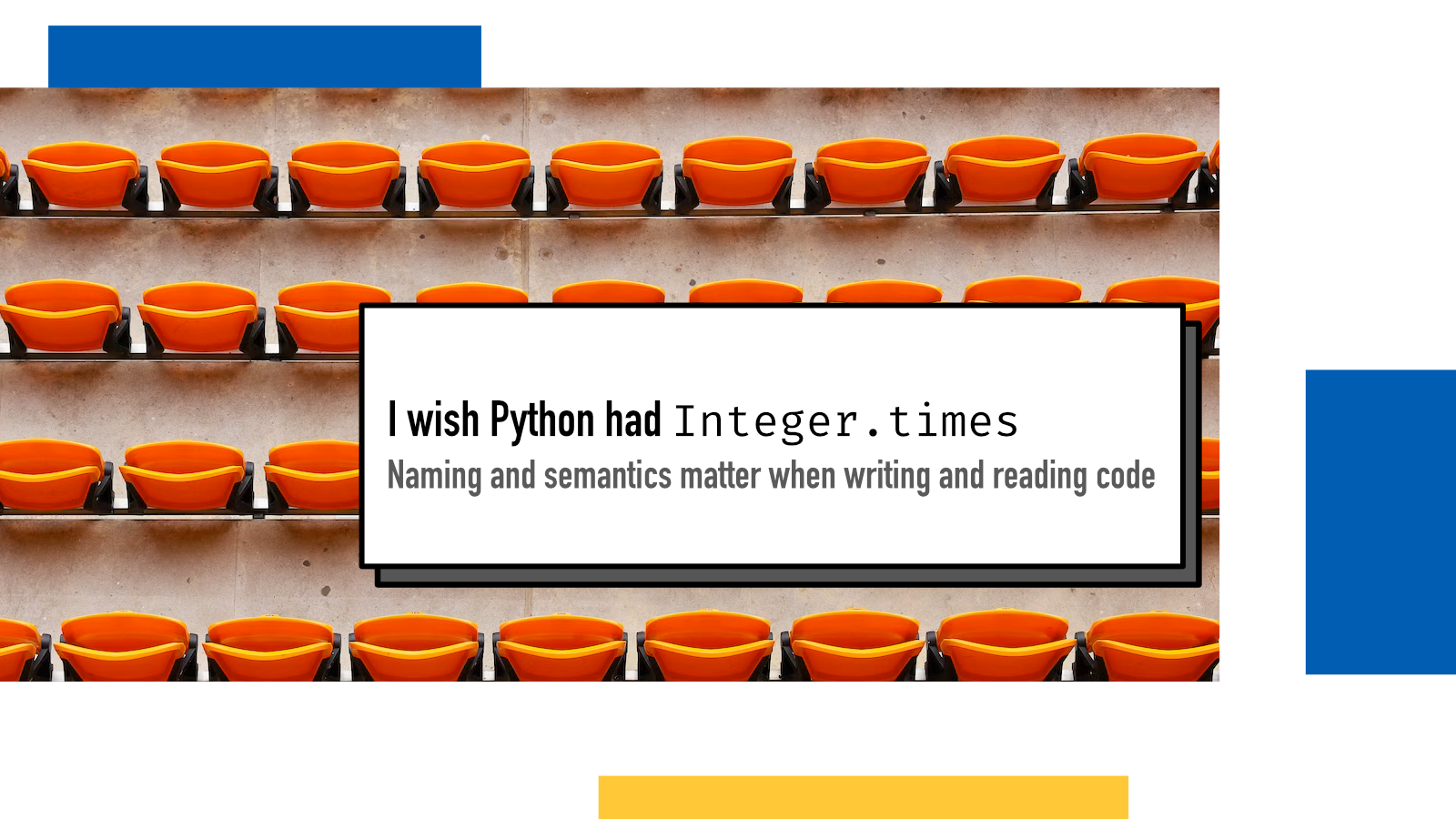
An old adage says that we read the code more than we write it. To make code more readable, we pay attention to variable and function naming and the available structures in the language. Sometimes we use the language long enough that we become used to certain mechanisms and how they work.
I was thinking about this last month when I was solving Advent of Code puzzles.
Looping n
times
In Ruby, integers have a method Integer#times that returns an Enumerator with values from 0 to n-1. This means we can do:
5.times { | _ | puts "This is printed five times" }
# or even
5.times { puts "This is printed five times" }
In Python, to achieve functionally similar result, we use
range
:
for _ in range(5):
print('This is printed five times')
When I write Python though, I often would like to be clearer that I’m
repeating something N times. Both
Integer#times
in Ruby and
range
in Python iterate over a range
from 0 to n-1 but they read very differently.
In Python, one could also use itertools.repeat which takes two arguments: object to return and how many times it is repeated:
from itertools import repeat
for _ in repeat(None, 5):
print('This is printed five times')
I do have a suspicion though that often this would get flagged in code review
and asked to switch to range
which saves
one import. And I guess most intermediate Python developers know how
range
work, making it understandable
enough.
A solution? Alias range
with
times
Every time I write for _ in range(n)
, I
wish there was an alias to use
for _ in times(n)
. I think it would make
it clearer when reading that here we don’t care about values, just how many
times the loop should be run.
I feel like this aliasing would be more pythonic than adding
times
method to
int
class. But it could also be that:
for _ in (5).times():
print('This is printed five times')
The difference between range
and
times
is not huge but I feel it’s
important. It conveys the message of what our code is doing. When we want to
iterate over a range, we use range
and
when we want to just repeat something, we use
times
.
If something above resonated with you, let's start a discussion about it! Email me at juhamattisantala at gmail dot com and share your thoughts. In 2025, I want to have more deeper discussions with people from around the world and I'd love if you'd be part of that.